Cube Exercise Files
This is the code for my cube, along with the image. You should be able to view the cube by going to:
http://arturtle.com/ALainez_Box.html
CODE:
<!DOCTYPE html>
<html>
<head>
<title>My first Three.js app</title>
<style>
body { margin: 0; }
canvas { width: 100%; height: 100% }
</style>
</head>
<body>
<script src="js/three.js"></script>
<script>
var scene = new THREE.Scene();
var camera = new THREE.PerspectiveCamera( 100, window.innerWidth/window.innerHeight, 0.1, 1000 );
var texture = THREE.ImageUtils.loadTexture('Supahsup.jpg')
var renderer = new THREE.WebGLRenderer();
renderer.setSize( window.innerWidth, window.innerHeight );
document.body.appendChild( renderer.domElement );
var geometry = new THREE.BoxGeometry( 30, 20, .4 );
var material = new THREE.MeshBasicMaterial( { map: texture } );
var cube = new THREE.Mesh( geometry, material );
scene.add( cube );
camera.position.z = 25;
var render = function () {
requestAnimationFrame( render );
cube.rotation.x -= 0.0017;
cube.rotation.y += 0.0045;
renderer.render(scene, camera);
};
render();
</script>
</body>
</html>
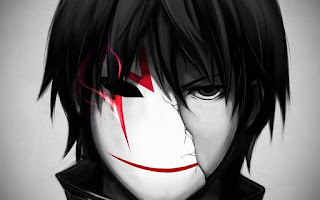
http://arturtle.com/ALainez_Box.html
CODE:
<!DOCTYPE html>
<html>
<head>
<title>My first Three.js app</title>
<style>
body { margin: 0; }
canvas { width: 100%; height: 100% }
</style>
</head>
<body>
<script src="js/three.js"></script>
<script>
var scene = new THREE.Scene();
var camera = new THREE.PerspectiveCamera( 100, window.innerWidth/window.innerHeight, 0.1, 1000 );
var texture = THREE.ImageUtils.loadTexture('Supahsup.jpg')
var renderer = new THREE.WebGLRenderer();
renderer.setSize( window.innerWidth, window.innerHeight );
document.body.appendChild( renderer.domElement );
var geometry = new THREE.BoxGeometry( 30, 20, .4 );
var material = new THREE.MeshBasicMaterial( { map: texture } );
var cube = new THREE.Mesh( geometry, material );
scene.add( cube );
camera.position.z = 25;
var render = function () {
requestAnimationFrame( render );
cube.rotation.x -= 0.0017;
cube.rotation.y += 0.0045;
renderer.render(scene, camera);
};
render();
</script>
</body>
</html>
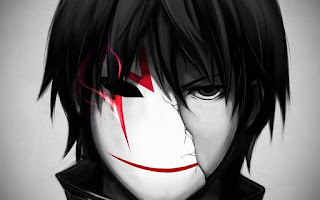
Comments
Post a Comment